How to URL Decode a String in PHP
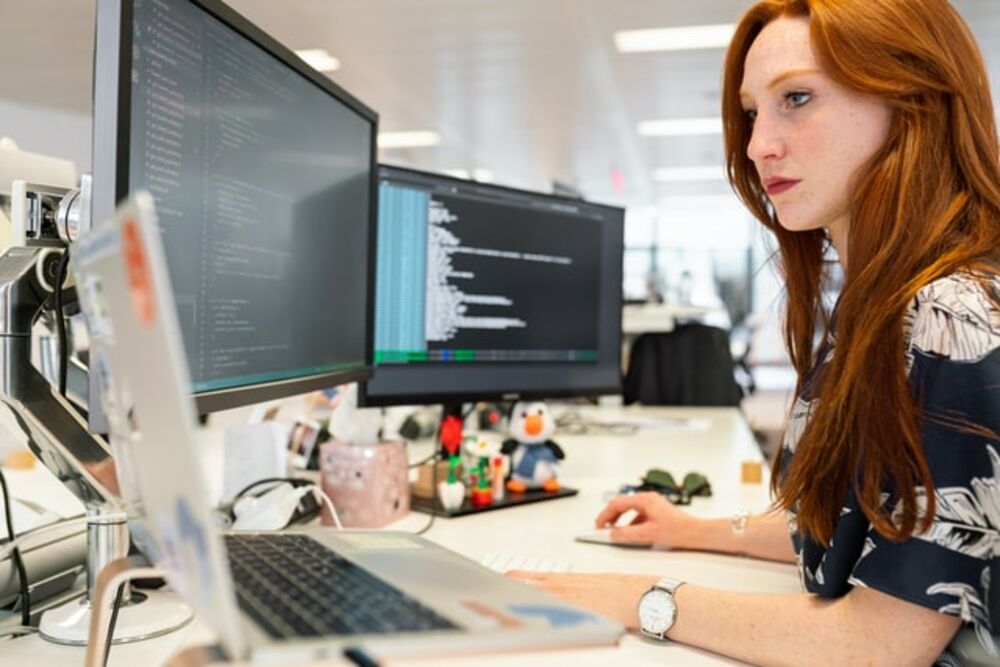
Introduction
URL decoding in PHP is an essential process for extracting and interpreting data accurately from URLs, especially when handling special characters, spaces, and non-alphanumeric characters. It plays a vital role in ensuring data integrity within web applications. In this guide, we will take you through the process of URL decoding a string in PHP, offering both code examples and detailed explanations.
URL Decoding in PHP: Code and Explanation
PHP provides a built-in function called urldecode()
for URL decoding strings. Here's how you can use it:
<?php // Encoded URL string $encodedString = "Hello+World%21+How+are+you%3F"; // URL decode the string $decodedString = urldecode($encodedString); // Display the decoded string echo "Decoded String: " . $decodedString . "<br>"; ?>
Explanation
-
Encoded URL String: Start with the encoded URL string that you want to decode. This string may contain encoded spaces, special characters, or non-alphanumeric characters.
-
URL Decoding: Utilize the
urldecode()
function to decode the encoded URL string. The function converts%20
back to spaces and decodes other percent-encoded characters to their original form. -
Display the Decoded String: Finally, you can display the decoded string by echoing it. The resulting string is now in its original form.
Example Output
For the encoded URL string "Hello+World%21+How+are+you%3F," the decoded string would appear as follows:
# Output Decoded String: Hello World! How are you?
The +
symbols have been replaced with spaces, %21
has been restored to an exclamation mark !
, and %3F
is back to being a question mark ?
.
URL decoding is crucial for accurately retrieving and processing data from URLs, making it a fundamental aspect of web development, particularly when handling user input and dynamic URLs.