How to URL Decode a String in Ruby
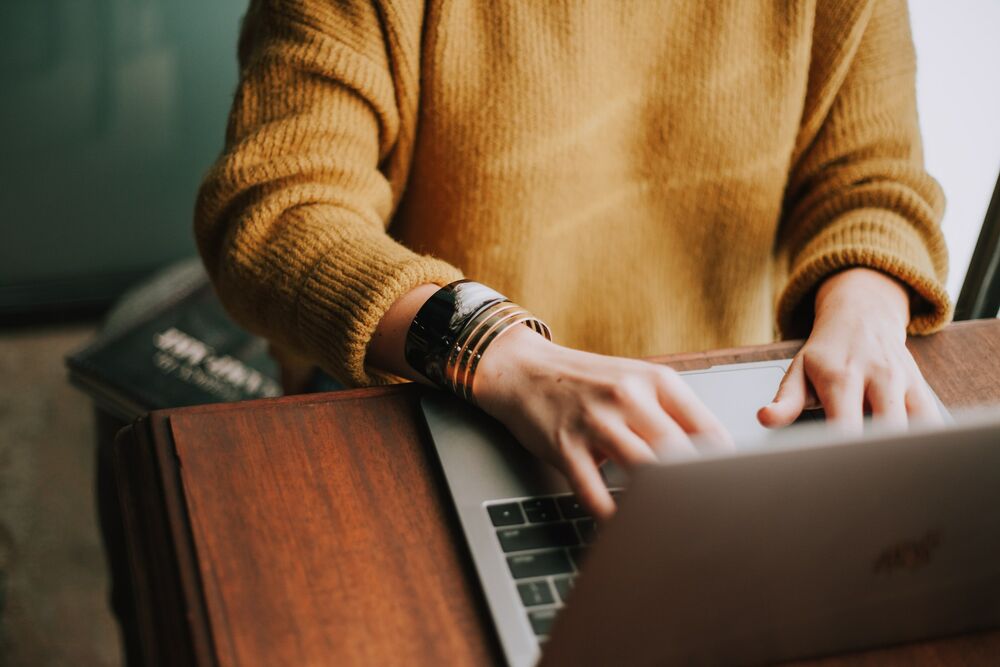
Introduction
URL decoding, also known as percent decoding, is a method used to safely interpret data in a URL by converting percent-encoded values back into their original reserved and special characters. In Ruby, you can perform URL decoding using the built-in URI
module, which offers the decode
method for this purpose.
URL Decoding in Ruby
To decode a URL-encoded string in Ruby using the URI
module, follow these steps:
require 'uri' # URL-encoded string encoded_string = "Hello,%20World!%20How%20are%20you%3F" # URL decoding decoded_string = URI.decode(encoded_string) puts "Encoded String: #{encoded_string}" puts "Decoded String: #{decoded_string}"
Explanation
-
Begin by importing the
URI
module withrequire 'uri'
. This module includes thedecode
method, essential for URL decoding. -
Define your URL-encoded string that you wish to decode.
-
Apply the
URI.decode
method to decode the URL-encoded string. This function transforms percent-encoded values back into their original reserved and special characters. -
Display both the encoded and decoded strings for verification.
Example Output
Encoded String: Hello,%20World!%20How%20are%20you%3F Decoded String: Hello, World! How are you?
In the output, you can observe that %20
has been decoded into spaces, and %3F
has been decoded into a question mark ?
. This ensures that the URL-encoded string is correctly interpreted, allowing you to work with the original data in a URL for various applications and web requests.