How to URL Decode a String in Python
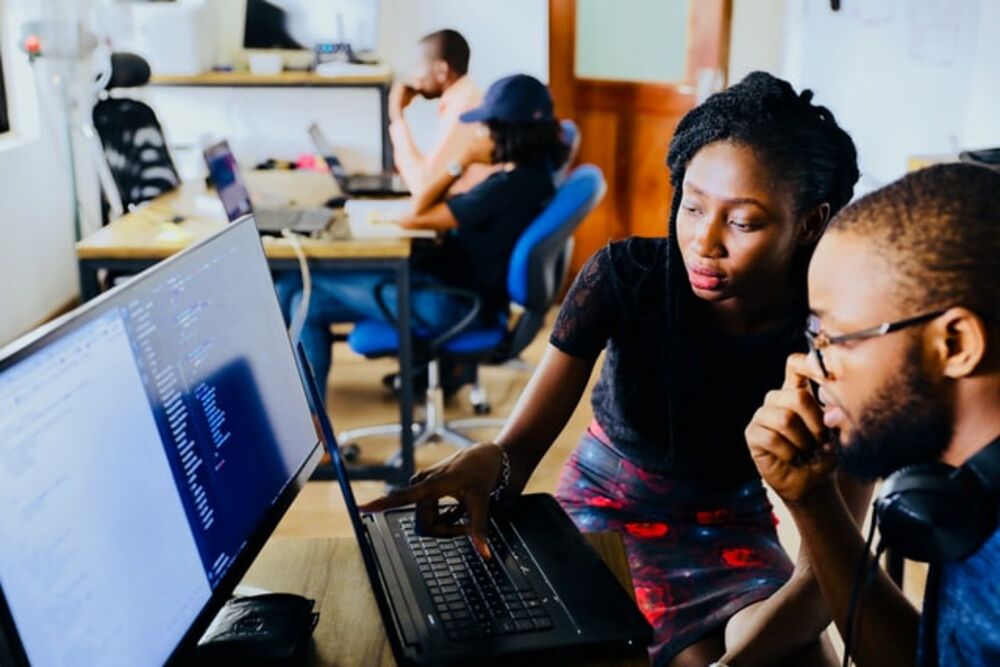
Introduction to URL Decoding
URL decoding, also known as percent decoding, is the process of converting percent-encoded characters in a URL or string back to their original form. In Python, the urllib.parse
module provides functions for URL decoding. This guide will demonstrate how to perform URL decoding in Python using the unquote()
function from the urllib.parse
module.
Code and Explanation
Here's an example of how to URL decode a string in Python:
import urllib.parse # URL-encoded string encoded_string = "Hello%2C%20World%21%20This%20is%20a%20test%20string%20with%20spaces%20and%20symbols%20like%20%40%20and%20%23%2E" # URL decode the string decoded_string = urllib.parse.unquote(encoded_string) # Print the encoded and decoded strings print("Encoded String:", encoded_string) print("Decoded String:", decoded_string)
Explanation
-
Import the
urllib.parse
module to access the URL decoding functions. -
Define the
encoded_string
variable, which contains the URL-encoded string you want to decode. This string includes percent-encoded representations of special characters, spaces, and symbols. -
Use the
urllib.parse.unquote()
function to URL decode theencoded_string
. This function replaces percent-encoded sequences with their original characters, restoring the string to its original form. -
Finally, print both the encoded and decoded strings to observe the transformation.
When you run this code, you'll notice that the decoded_string
contains the URL-decoded version of the encoded_string
. Percent-encoded characters such as %2C
, %20
, %21
, and %23
have been reverted to their original characters, making the string readable and usable.
URL decoding is essential when processing data from URLs or handling data received from web requests, ensuring that the data is correctly interpreted and used in Python applications.