How to URL Decode a String in Java Script
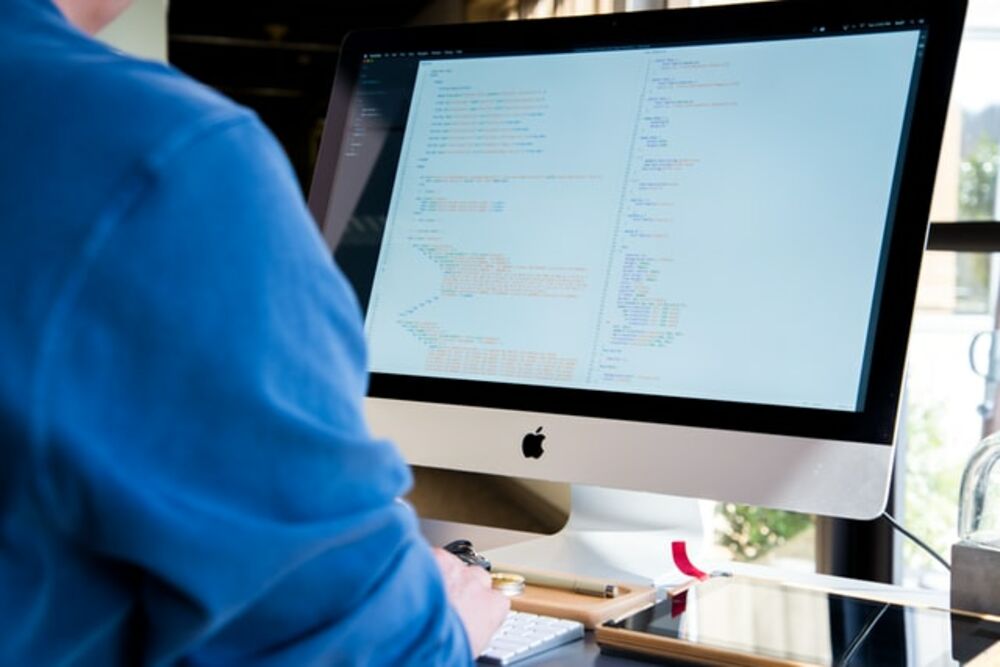
Introduction
URL decoding in JavaScript is a crucial skill for web developers as it allows you to extract data from URLs safely, ensuring accurate data retrieval. In this guide, we'll delve into how to decode a URL-encoded string in JavaScript and grasp the underlying principles.
URL Decoding in JavaScript
URL decoding can be accomplished using the decodeURIComponent()
function in JavaScript. This function takes a URL-encoded string as input and returns the original string by replacing percent-encoded values with their respective characters.
Example Code:
// URL-encoded string with special characters const encodedString = "Hello%2C%20World%21%20This%20is%20a%20test%2E"; // Decoding the URL-encoded string const decodedString = decodeURIComponent(encodedString); console.log("URL-encoded String:", encodedString); console.log("Decoded String:", decodedString);
Explanation
-
We start with the
encodedString
, which contains URL-encoded values such as%20
for spaces,%2C
for commas, and%21
for exclamation marks. -
We use
decodeURIComponent(encodedString)
to decode the URL-encoded string. This function replaces percent-encoded values with their original characters. -
Finally, we print both the URL-encoded and decoded strings to the console for comparison.
Conclusion
URL decoding is a fundamental technique in web development, ensuring accurate data retrieval from URLs. By utilizing decodeURIComponent()
in JavaScript, you can confidently extract data from your web applications, maintaining data integrity and proper URL handling.