How to URL Decode a String in Golang
2 Mins 08/06/2023
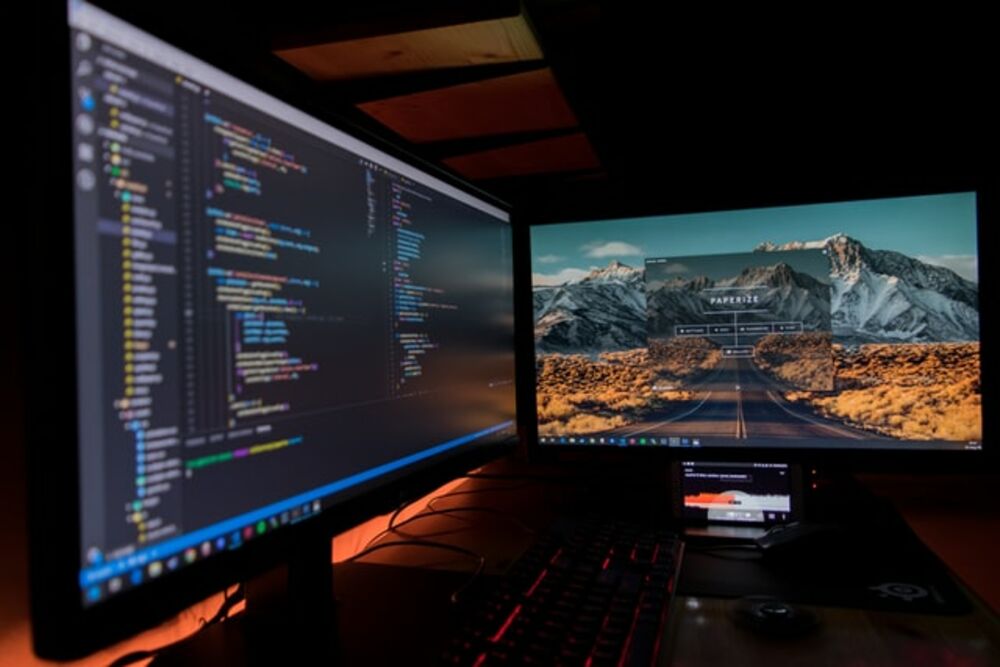
Introduction
URL decoding is a fundamental operation in web development that allows you to extract meaningful information from URLs, including special characters and spaces. In Go, the net/url
package provides functions for URL decoding. This guide will demonstrate how to perform URL decoding in Go, complete with code examples and detailed explanations.
Code and Explanation
- Begin by importing the
net/url
package to access the URL decoding functions.
import ( "net/url" "fmt" )
- Define the URL-encoded string that you want to decode.
encodedString := "Hello%2C%20World%21%20How%20are%20you%3F"
- Utilize the
url.QueryUnescape()
function to decode the URL-encoded string. This function replaces percent-encoded values with their corresponding characters.
decodedString, err := url.QueryUnescape(encodedString) if err != nil { fmt.Println("Error decoding:", err) return }
- Finally, print the decoded string to see the result.
fmt.Println("Decoded URL:", decodedString)
Full Code Example:
Here's the complete Go code for URL decoding a string:
package main import ( "net/url" "fmt" ) func main() { encodedString := "Hello%2C%20World%21%20How%20are%20you%3F" decodedString, err := url.QueryUnescape(encodedString) if err != nil { fmt.Println("Error decoding:", err) return } fmt.Println("Decoded URL:", decodedString) }
Explanation:
- We import the
net/url
package to access theurl.QueryUnescape()
function. - The
encodedString
contains the URL-encoded string that we want to decode. - We use
url.QueryUnescape()
to decode theencodedString
, and any potential decoding errors are handled. - Finally, we print the
decodedString
, which now represents the URL-decoded version of the original string.
By following these steps, you can safely perform URL decoding in Go, allowing you to extract meaningful data from URLs in your web applications.