How to URL Decode a String in Java
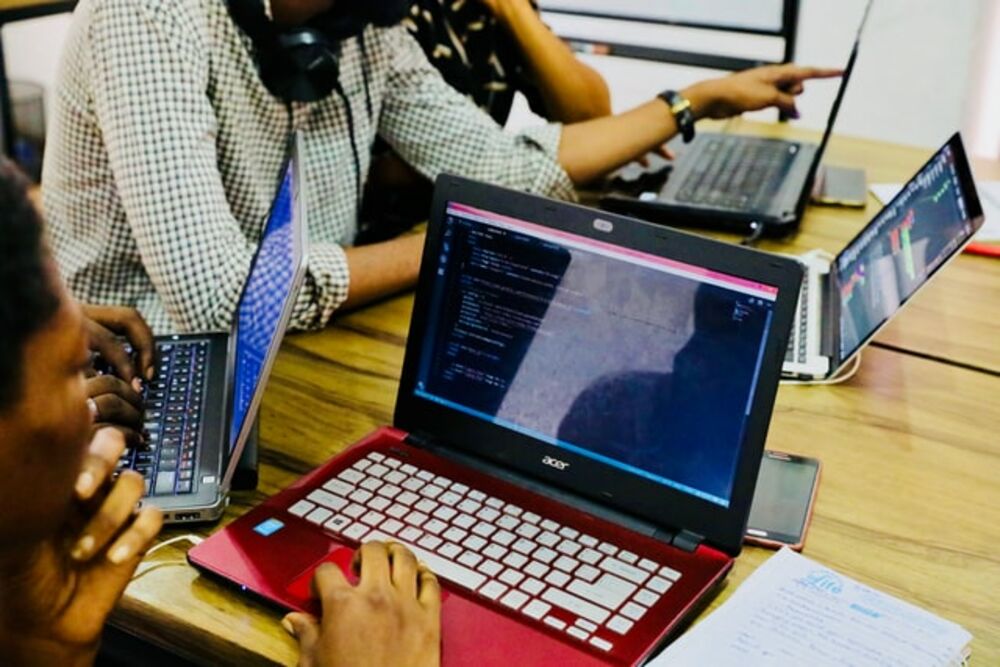
Introduction
URL decoding is a vital task in Java when you need to safely extract data from a URL. It ensures that encoded characters in the URL are correctly represented and can be converted back to their original form. In Java, you can perform URL decoding using the URLDecoder
class from the java.net
package.
Code and Explanation
Here's how to URL decode a string in Java:
import java.io.UnsupportedEncodingException; import java.net.URLDecoder; public class URLDecodingExample { public static void main(String[] args) { try { // Encoded URL string String encodedString = "Hello%2C+World%21"; // URL decode the string String decodedString = URLDecoder.decode(encodedString, "UTF-8"); // Print the decoded string System.out.println("Decoded Text: " + decodedString); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } } }
Explanation:
-
Import the necessary classes:
java.io.UnsupportedEncodingException
for handling decoding errors.java.net.URLDecoder
for decoding the URL-encoded string.
-
Create a
main
method for your Java program. -
Define the encoded URL string from which you want to extract data.
-
Use
URLDecoder.decode()
to decode the encoded URL string. The second parameter,"UTF-8"
, specifies the character encoding scheme used during encoding (UTF-8 is a common choice). -
Print the decoded string to the console.
This code illustrates how to perform URL decoding in Java, ensuring that the encoded URL string is correctly converted back to its original form, allowing you to extract meaningful data from it.